Improving the injector from last post to hopefully bypass AV
Environment Setup
OS: Windows 10
Target: Running processes with the same integrity level
These are some tools that might be useful.
- API Monitor: http://www.rohitab.com/apimonitor
- Process Hacker: https://processhacker.sourceforge.io/
The previous injector is still pretty detectable by static analysis, so let’s make it more stealthy this time.
Methodology
- Remove debugging symbols and statements
- Remove strings that hint anything malicious
- Generate shellcode exits gracefully without crashing program
- XOR encrypt shellcode and decode during runtime
- “Predict” if being executed by AV and change behaviour
As usual, coding in the C language because C++ is nasty.
Better shellcode
In the previous post we generated a shellcode with the following command.msfvenom -p windows/x64/exec CMD=cmd.exe -f c
When this shellcode exits, it ends the entire program, which is pretty noisy.
We can change this by using the EXITFUNC
flag.msfvenom -p windows/x64/exec CMD=cmd.exe EXITFUNC=thread -f c
Now when the shellcode exits, it will only terminate the thread that we spawn, and the original program will still be running.
XOR encrypt and decrypt
Cleartext msf shellcode is always a giveaway. We can make it better by XOR encrypting it.
Encryptor/Decryptor
1 |
|
Comments are self explanatory. The encrypt function is essentially also the decrypt function because A=(A^B)^B
Main function just formats encrypted shellcode nicely so we can copy and paste, and we only need the encrypt function in our actual injector.
“Predict” AV execution
So apparently AVs will try to “speed up” your program if they detect a sleep in it, so it won’t spend too long analysing. We can abuse this by checking if our program was indeed “sped up”.
1 | time_t cur = time(0); |
Just a simple check that quits the program if it “predicts” an AV executing it. Delays execution by 15 seconds so some AV might even give up analysing it lol. Patience is key.
Remove strings and debug symbols
Running the command strings
on our exe reveals strings such as “NtCreateThreadEx”, which may lead to AV detection.
We can remove these strings by again XOR encrypting them and decrypting during runtime.
For example:
1 | unsigned char * ntdll = decrypt("\x3d\x27\x37\x3f\x3f\x7d\x37\x3f\x3f\x53", 10, 0x53); |
Repeat this process until all abnormal strings are removed.
To remove debug symbols, just run strip <program.exe>
Final
1 |
|
Let’s use antiscan.me to check detection rate.
Before:
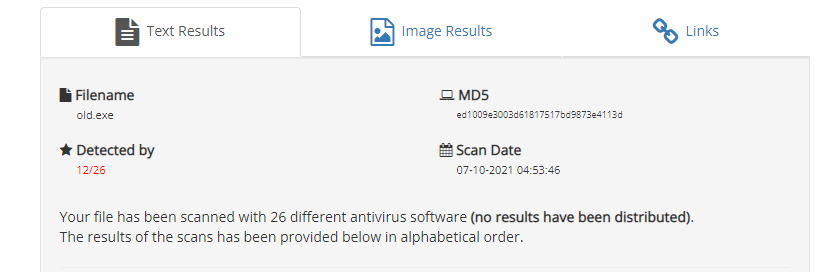
After:
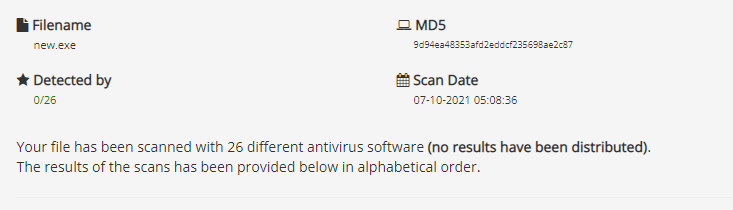
Pretty neat!
Conclusion
Still a pretty rudimentary bypass as we didn’t take into account hooks and AMSI and all the improved monitoring tools out there.
AV manufacturers and hackers have played years of cats and mice, and there are lots to learn :)